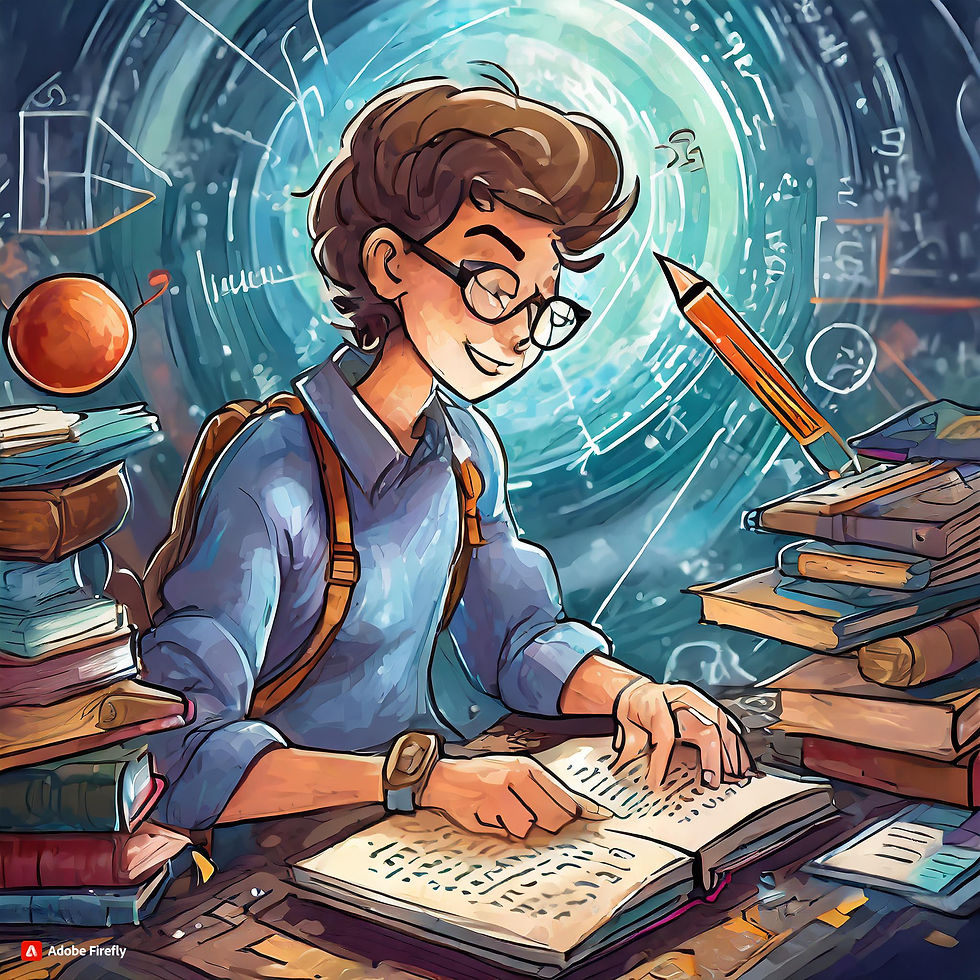
(S) Single responsibility principle
Single responsibility principle (SRP) states that each entity should be responsible for only one job or solve only one problem
Let’s consider an example of how we usually write a code WITHOUT following SRP:
This breaks our SRP since we’re performing all the actions inside the Handler entity itself which should be split up in different entities of its own and handled separately. Now, let’s see how the code looks like with following SRP:
SRP benefits us in the following manner:
Higher cohesion
Reduced chances of code duplication
Reduced bloated classes
Easier to test and maintain
Keeps the code clean as multiple responsibilities are now segregated
(O) Open-Closed principle
Open-Closed principle (OCP) states that an entity should be open for extension but closed for modification. This means that a class should be easily extendable without having to modify the existing code
The definition sounds contradictory at first but once you start following it at scale, it will make a lot more sense. To really narrow this principle down: You can create classes and extend them (by creating a subclass), but you can’t modify the original class. Reason for doing so can be :
Consider someone else wrote a class and you need to add functionalities to it or add a change to it, you’d constantly need to ask the original author to change it for you, or you’d need to dive into it to change it yourself. Now, the original author might have different thought process for all the code they wrote that got the feature working
Even more, the class would start to incorporate(or simply add) many different concerns(or simply functionalities), which might break SRP
Consider the above SRP enabled example from above. In that, we were saving the person object to UserDefaults but now we want to save to CoreDataas well. Our go-to approach would be:
This breaks the OCP since we’re changing the original implementation of save-to-User-Defaults that has been thoroughly tested and later deployed. To accommodate OCP, we can make use of either Inheritance via classes or simply use protocol conformance which all of us Swift developers love to use!
If you don’t want to use protocol conformance, you can always use inheritance via classes to achieve the same:
Benefits of OCP can be as follows:
Code is easy to maintain and review
Encourages the use of abstraction and polymorphism
Helps us to reduce potential bugs since it does not allow us to modify the existing code which is already tested and ready to deploy
(L) Liskov substitution principle
To be honest, Liskov substitution principle(LSK) is arguably one of the most confusing principles of all, at first !
What it essentially states is that objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program. LSK is simply inheritance with additional step
It’ll be hard and confusing to use the above stated examples to explain LSK so I’ll use a different, simple example. Also, I’ll not be using Rectangle and Square example as the internet is already flooded with those and at times it becomes confusing
So how do we resolve this? We create a separate class for birds that can fly:
Benefits of LSP includes:
Code remains flexible and modular
Reduces code duplication
(I) Interface segregation principle
Interface segregation principle(ISP) states that a client should never be forced to implement an interface that it doesn’t use, or clients shouldn’t be forced to depend on methods they do not use. To put it in simple words, instead of using one fat interface(or protocol), many small interfaces are preferred based on methods the provide
ISP might sound similar to SRP but SRP usually applies to structs/classes and ISP applies to protocols
We can use the Bird example above to explain ISP; however we will be using protocol(or interface in other languages) so that our entity can conform to more than one protocols:
To resolve this, we break our Bird protocol into sub protocols to accommodate each method:
Benefits of ISP includes:
More focused and cohesive interfaces
Avoid unnecessary dependencies
Avoid single bloated interface
Promotes decoupling
(D) Dependency Inversion principle
Dependency Inversion principle(DIP) states that high-level modules should not depend on low-level modules; both should depend on abstractions or simply put: Entities must depend on abstractions, not on concretions
This ensures that code is relying on the smallest possible surface area — in fact, it doesn’t depend on code at all, just a contract defining how that code should behave. Doing so also ensures that if some piece of code breaks at one place, it does not create a cascading effect of code breaking at other dependent places
DIP sounds pretty similar to OCP so its example can also serve as an example for DIP. However, I’ll use a different example here:
The above example breaks DIP since there’s a lot of tight coupling and Payment class which is a high-level module is dependent on CreditCardPayment which is a low-level module. As per DIP, it should depend on abstraction/protocol
Benefits of DIP includes:
Encourages decoupling
Makes code more flexible, agile, and reusable
Promotes the use of interfaces or protocols to define contracts between components
To sum it all up, there are a lot of benefits of incorporating SOLID principles in your app:
Enables us to write code that is easier to understand, maintain and extend
The lesser the responsibilities on an entity, the fewer the chances of breaking our code when making changes to an entity
Enables us to write more scalable and testable code
Helps us to refactor the code with fewer complications
Helps us to use just the right level of coupling — keep things together that belong together, and keep them apart if they belong apart
That’s it, folks! Happy Coding!
Commenti